In THIS ARTICLE, I will show you my experiments using Bluetooth to exchange data between my phone and EPS32 to perform various functions. This project is part of a course in embedded systems guided by the excellent Dr. Kusprasapta Mutijarsa, S.T, M.T. of the Bandung Institute of Technology (ITB).
Prerequisites
- An ESP32 microcontroller, along with its vertebrate
- A breadboard
- A Micro-USB cord
- A few male-to-male jumper wires
- A few male-to-female jumper wires
- A few 3mm LEDs
- A few resistors
- A phone
- Arduino IDE installed for ESP32. I have made a detailed installation guide in my previous article: https://zhillan-arf.medium.com/blink-the-led-with-esp32-5c3f358ea2ff
Bluetooth
Bluetooth is one of the many ways devices can communicate wirelessly. There exist two types of Bluetooth: Classic (or Basic Rate) and Low Energy, with the latter being approximately 100 times more energy-efficient than the former. In general, these are the differences between them.
Using Bluetooth to remotely control LEDs and send data to the phone
I started with Bluetooth Classic, which is relatively simpler. Bluetooth function is already inside the microcontroller, indeed the Arduino IDE that I used already has an example code called SerialToSerialBT.

This example program will establish a two-way serial Bluetooth communication between two devices (one of them being the microcontroller).
#include "BluetoothSerial.h"#if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED)
#error Bluetooth is not enabled! Please run `make menuconfig` to and enable it
#endifBluetoothSerial SerialBT;void setup() {
Serial.begin(115200);
SerialBT.begin("dialah_zhillanku.bt"); //Bluetooth device name
Serial.println("'dialah_zhillanku.bt' started, now you can pair it with bluetooth!");
}void loop() {
if (Serial.available()) {
SerialBT.write(Serial.read());
}
if (SerialBT.available()) {
Serial.write(SerialBT.read());
}
delay(20);
}
I compiled and ran the program, and this was the result displayed in the serial monitor.

As you can see from the program, it will broadcast a Bluetooth port that can be detected by other devices (I named it dialah_zhillanku.bt), namely my phone. I used the Serial Bluetooth Terminal app on Google Playstore as my primary interface.

As you can see, dialah_zhillanku.bt is detected and displayed by the phone’s Bluetooth interface. We can use the app to send messages to the serial monitor.


It was a success!
Next, I used this Bluetooth connection to turn on a LED in ESP32, using this contraption.
I used this program. In this program, SerialBT reads Bluetooth message transmissions per character, and switches the LED outlet pin on and off depending on the message.
#include “BluetoothSerial.h”// Check if Bluetooth configs are enabled
#if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED)
#error Bluetooth is not enabled! Please run `make menuconfig` to and enable it
#endif// Bluetooth Serial object
BluetoothSerial SerialBT;// GPIO where LED is connected to
const int ledPin = 5;// Handle received and sent messages
String message = “”;
char incomingChar;void setup() {
pinMode(ledPin, OUTPUT);
Serial.begin(115200);
// Bluetooth device name
SerialBT.begin(“ESP32”);
Serial.println(“The device started, now you can pair it with bluetooth!”);
}void loop() {
unsigned long currentMillis = millis();
// Read received messages (LED control command)
if (SerialBT.available()){
char incomingChar = SerialBT.read();
if (incomingChar != ‘\n’){
message += String(incomingChar);
}
else{
message = “”;
}
Serial.write(incomingChar);
}
// Check received message and control output accordingly
if (message ==”led_on”){
digitalWrite(ledPin, HIGH);
}
else if (message ==”led_off”){
digitalWrite(ledPin, LOW);
}
delay(20);
}
Here is the result.
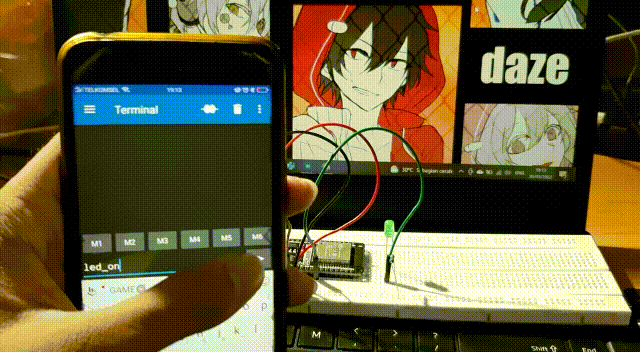
Bluetooth Low Energy
As the name suggests, energy is the big factor behind Bluetooth LE, which apparently uses 100 times less power than classic Bluetooth. This is BLE is always on a sleep state until an action is initiated.
Now, I created a contraption and program that performs the same functions as the previous. The program is as follows.
#include <BLEDevice.h>
#include <BLEUtils.h>
#include <BLEServer.h>#define CHARACTERISTIC_UUID "beb5483e-36e1-4688-b7f5-ea07361b26a8"
#define SERVICE_UUID "4fafc201-1fb5-459e-8fcc-c5c9c331914b"void setup() {
Serial.begin(115200);
Serial.println("Starting BLE work!");
BLEDevice::init("dialah_zhillanku.ble");
BLEServer *pServer = BLEDevice::createServer();
BLEService *pService = pServer->createService(SERVICE_UUID);
BLECharacteristic *pCharacteristic = pService->createCharacteristic(
CHARACTERISTIC_UUID,
BLECharacteristic::PROPERTY_READ |
BLECharacteristic::PROPERTY_WRITE
);
pCharacteristic->setValue("Hello, client!");
pService->start();
BLEAdvertising *pAdvertising = BLEDevice::getAdvertising();
pAdvertising->addServiceUUID(SERVICE_UUID);
pAdvertising->setScanResponse(true);
pAdvertising->setMinPreferred(0x06); // functions that help with iPhone connections issue
pAdvertising->setMinPreferred(0x12);
BLEDevice::startAdvertising();
Serial.println("Characteristic defined! Now you can read it in your phone!");
}
void loop() {
delay(2000);
}
This code sets up the ESP32 as the server, and will send the string “Hello, client!”. After compiling and running the program, I then created client using the nRF Connect for Mobile app on my phone.

When I opened the client and connect it to the board serrver, it was exactly what I wanted it to be: the message “Hello, client!” successfully transferred from the server!
